To require users of your site to authenticate, you simply need to place some instructions into your Web.Config file. (You may edit the file directly, or you may use a tool such as the Web Site Administration tool available through Visual Studio.)
Web.Config has a section for specifying how your site should deal with authentication and authorization. In the absence of the authentication and authorization elements, ASP.NET allows unrestricted access to your site. However, once you add these elements to your Web.Config file ASP.NET will force a redirect to a file you specify. Most of the time, the file will be some sort of login page where users must do something such as type in a user name and password.
Before looking at the code, take a look at Figure 10-2, which illustrates how control flows on your Web site when you turn on Forms Authentication using Web.Config.
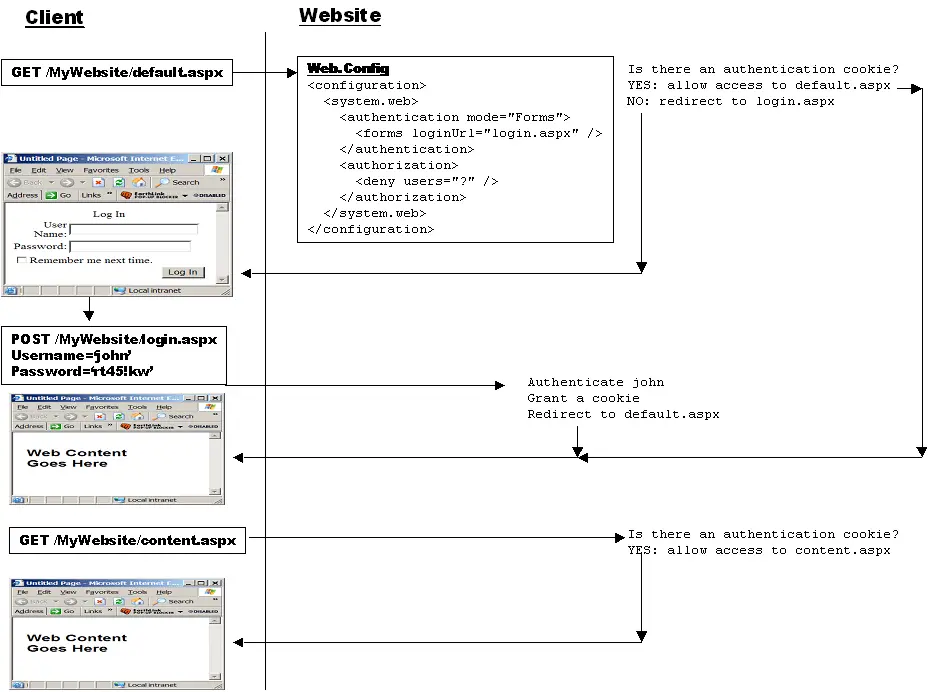
Figure 10-2 The control flow for a site with Forms Authentication turned on.
To see an example of the most basic authentication you can use in your application, take a look at the files Login.aspx and Web.ConfigFormsAuthentication. The Web.Config includes the Authentication and Authorization elements to support Forms Authentication for the site. Listing 10-1 shows the Web.Config to force authentication.
Listing 10-1
<configuration> <system.web> <authentication mode="Forms"> <forms loginUrl="login.aspx" /> </authentication> <authorization> <deny users="?" /> </authorization> </system.web> </configuration>
The login page that goes with it is shown in Listing 10-2.
Listing 10-2
<%@ Page language=C# %> <html> <script runat=server> protected bool AuthenticateUser(String strUserName, String strPassword) { if (strUserName == "Gary") { if(strPassword== "K4T-YYY") { return true; } } if(strUserName == "Jay") { if(strPassword== "RTY!333") { return true; } } if(strUserName == "Susan") { if(strPassword== "erw3#54d") { return true; } } return false; } public void OnLogin(Object src, EventArgs e) { if (AuthenticateUser(m_textboxUserName.Text, m_textboxPassword.Text)) { FormsAuthentication.RedirectFromLoginPage( m_textboxUserName.Text, m_bPersistCookie.Checked); } else { Response.Write("Invalid login: You don't belong here…"); } } </script> <body> <form runat=server> <h2>A most basic login page</h2> User name: <asp:TextBox id="m_textboxUserName" runat=server/><br> Password: <asp:TextBox id="m_textboxPassword" TextMode="password" runat=server/> <br/> Remember password and weaken security?: <asp:CheckBox id=m_bPersistCookie runat="server"/> <br/> <asp:Button text="Login" OnClick="OnLogin" runat=server/> <br/> </form> </body> </html>
This is a simple login page that keeps track of three users-Gary, Jay, and Susan. Of course, in a real application this data would come from a database rather than being hard-coded into the page.
In this scenario, even if users try to surf to any page in the virtual directory, ASP.NET will stop them dead in their tracks and force them to pass the login page shown in Figure 10-3.
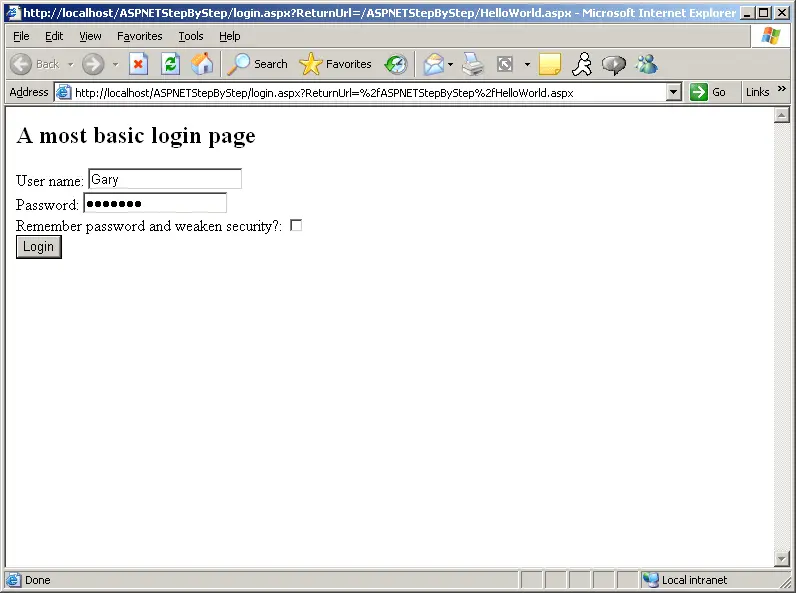
Figure 10-3 A simple login page for getting a user name and password from a client.
This simple login page authenticates the user (out of a group of three possible users). In a real Web site, the authentication algorithm would probably use a database lookup to see if the user identifying himself or herself is in the database and whether the password matches. Later in this tutorial, we'll see the ASP.NET 2.0 authentication services. The login page then issues an authentication cookie using the FormsAuthentication utility class.
Here's what the Web page looks like in the browser with tracing turned on. Here you can see the value of the Authentication cookie in the cookie collection.
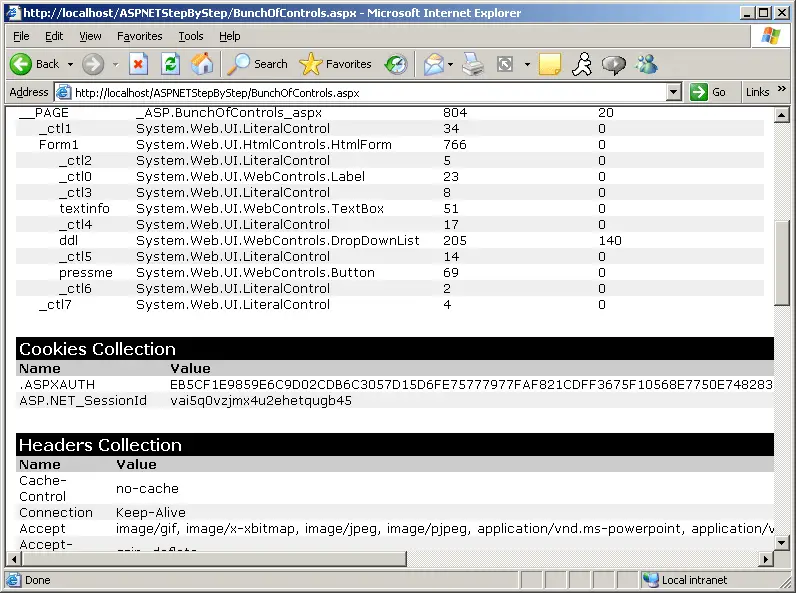
Figure 10-4 Tracing turned on reveals the authentication cookie for a page using Forms Authentication.
Run the Forms Authentication Example
-
This example shows how to employ Forms Authentication on your site.
To run the Forms Authentication example, create a virtual directory to hold the site. Alternatively, you can use an already existing site and employ Forms Authentication there.
-
Copy the Login.aspx page from the Tutorial 10 examples into the virtual directory for which you want to apply Forms Authentication.
-
Copy the Web.ConfigForceAuthentication file from the Tutorial 10 examples into the virtual directory for which you want to apply Forms Authentication. Make sure the configuration file is named Web.Config after you copy it.
-
Try to surf to a page in that virtual directory. ASP.NET should force you to complete the Login.aspx page before moving on.
-
Type in a valid user name and password. Subsequent access to that virtual directory should work just fine because now there's an Authentication ticket associated with the request and response.
While you may build your own authentication algorithms, ASP.NET 2.0 includes a number of new features that make authenticating users a straightforward and standard proposition. We'll look at those in a moment.
Briefly, ASP.NET allows two other types of authentication: Passport authentication and Windows authentication. Passport authentication relies upon Passport-a centralized authentication service provided by Microsoft. If you've ever used hotmail.com, you've used Passport. The advantage of Passport authentication is that it centralizes login and personalization information at one source.
The other type of authentication supported by ASP.NET is Windows authentication. If you specify Windows authentication, ASP.NET relies upon IIS and Windows authentication to manage users. Any user making his or her way through IIS authentication (using basic, digest, or Integrated Windows Authentication as configured in IIS) will be authenticated for the Web site. These other forms of authentication are available when configuring IIS. However, for most ASP.NET Web sites, you'll be bypassing IIS authentication in favor of ASP.NET authentication. ASP.NET will use the authenticated identity to manage authorization.