This tutorial covers the following topics:
PHP 5.3.0 introduced the goto statement, which performs a jump to a specified label (line) within the same file. A label is a name followed by a colon :
.
<?php $i = 5; if ($i < 10) goto jump; //This will be jumped echo 'BrainBell.com'; //label jump: echo 'This should be printed.';
Create a goto loop
<?php $i = 0; REPEAT: if ($i < 10) { $i++; echo "$i "; goto REPEAT; } echo '<br>Finished goto loop';
The above example shows the following result on the web browser window:
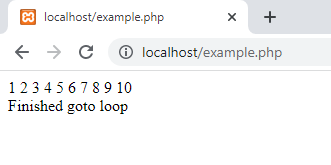
Use goto
inside function
The target label must be within the same script file and scope. You cannot jump out of a function or method, nor can you jump into one.
<?php function abc(){ //some code goto jump; } abc(); //... jump: echo '....';
The above example prints the following fatal error:
PHP Fatal error: 'goto' to undefined label "jump"...
Use goto
inside a loop
<?php $resultsToFetch = 100; $resultsFetched = 0; FetchMore: $results = rand(5,20); while (true){ $resultsFetched += $results; if ($resultsFetched < $resultsToFetch) goto FetchMore; break; } echo $resultsFetched;
PHP Control Structures: