- Specifying timezone to get the date & time of different countries
- Get latitude, longitude, and country code for a timezone
- Get the timezone offset from UTC (GMT)
- Get previous and upcoming Daylight Saving date/time information for a specific timezone
- Get time zone information by time zone abbreviations
- Get a list of supported timezone identifiers in PHP
The DateTimeZone class works with the DateTime class. The DateTime constructor takes an optional second argument, which is a DateTimeZone instance. The PHP’s default time zone applies if the second argument is not provided.
Example: Specifying timezone in the constructor
To get the date and time of a specific time zone, pass a valid timezone identifier into the DateTimeZone class constructor:
<?php $timezone = new DateTimeZone('Australia/Adelaide'); $date = new DateTime('now', $timezone); echo $date->format('d M Y, H:i:s') . '<br>'; # 13 Aug 2022, 13:44:31
If you omit the constructor’s second argument, the time zone is determined by PHP’s default timezone setting:
<?php // No DateTimeZone instance $date = new DateTime('now'); echo $date->format('d M Y, H:i:s') . '<br>'; # 18 Aug 2022, 15:16:29 $timezone = new DateTimeZone('Australia/Adelaide'); $date = new DateTime('now', $timezone); echo $date->format('d M Y, H:i:s'); # 18 Aug 2022, 22:46:29
Example: DateTime::setTimezone() method to specify timezone later
You can specify or change the time zone of a DateTime object later by passing a DateTimeZone object as an argument to the setTimezone()
method:
<?php $timezone = new DateTimeZone('Australia/Adelaide'); $date = new DateTime('now', $timezone); echo $date->format('d M Y, H:i:s') . '<br>'; # 13 Aug 2022, 13:44:31 $timezone = new DateTimeZone('America/Eirunepe'); $date->setTimezone($timezone); echo $date->format('d M Y, H:i:s'); # 12 Aug 2022, 23:14:31
Example: DateTime::getTimezone() method to get timezone information
The getTimezone()
method returns a DateTimeZone object representing the DateTime object’s time zone:
<?php $date = new DateTime(); $timezone = $date->getTimezone(); echo $timezone->getName(); # Prints: Europe/Berlin
DateTimeZone::getLocation
How to get location information for a timezone?
You can get the location information from the time zone object using the getLocation()
method. This method returns an associative array that provides the country code of origin of the time zone, longitude & latitude, and some comments.
<?php $timezone = new DateTimeZone('Asia/Kolkata'); $location = $timezone->getLocation(); foreach ($location as $k => $v){ echo "$k: $v <br>"; } # country_code: IN # latitude: 22.53333 # longitude: 88.36666 # comments:
Use the following code if you want to retrieve location information from a DateTime object:
<?php $date = new DateTime(); $timezone = $date->getTimezone(); $location = $timezone->getLocation(); foreach ($location as $k => $v){ echo "$k: $v <br>"; } # country_code: DE # latitude: 52.5 # longitude: 13.36666 # comments: Germany (most areas)
You can also get the name of the timezone by using the getName()
method:
<?php $date = new DateTime(); $timezone = $date->getTimezone(); echo $timezone->getName(); # Europe/Berlin
DateTimeZone::getOffset
How to get the timezone offset from UTC (GMT)
The getOffset()
method returns the offset from UTC (Universal Coordinated Time) in seconds:
<?php $date = new DateTime(); $timezone = new DateTimeZone('Asia/Kolkata'); $offset = $timezone->getOffset($date); echo $offset . ' seconds <br>'; # 19800 seconds echo $offset / 3600 . ' hour(s)'; # 5.5 hour(s)
DateTimeZone::getTransitions
How to get all transitions for the timezone?
The getTransitions()
method returns a multidimensional array containing historical and future dates and times of switching to and from daylight saving time. The returned array structure is:
ts
key: Unix timestamptime
key: time stringoffset
key: UTC offset in secondsisdst
key: True if daylight saving time is active, false otherwise.abbr
key: Timezone abbreviation
<?php $timezone = new DateTimeZone('Asia/Karachi'); $transitions = $timezone->getTransitions(); print_r($transitions);
The above code prints the following result:
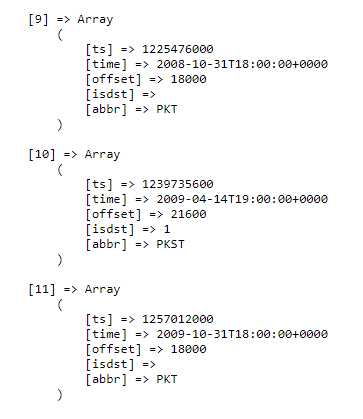
The result enables you to calculate whether daylight saving time is in force at a specific date and time. You can limit the range of results by providing the two optional timestamps: $timestampBegin
and $timestampEnd
, see the following example:
<?php $timestampBegin = strtotime(' 01 jan 2005'); $timestampEnd = strtotime ('01 jan 2015'); $timezone = new DateTimeZone('Asia/Karachi'); $transitions = $timezone->getTransitions( $timestampBegin, $timestampEnd); print_r($transitions);
The following output prints on the browser:
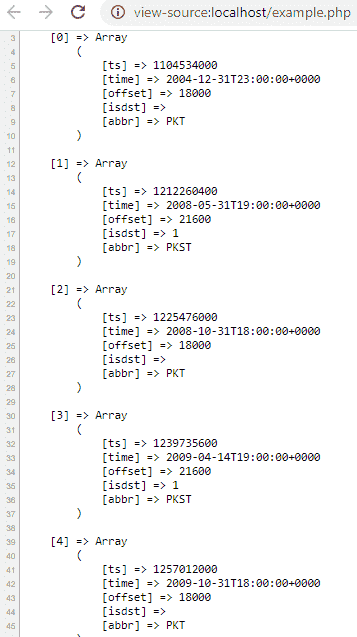
DateTimeZone::listAbbreviations
How to get DST, UTC offset, and the timezone name.
The listAbbreviations()
is a static method, it returns a multidimensional array. The array lists dst (daylight saving time), offset (UTC offset in seconds), and timezone_id for each time zone (e.g. GMT, EDT, EST etc.):
<?php $all_timezones = DateTimeZone::listAbbreviations(); print_r($all_timezones); # Prints all abbreviations $edt = $all_timezones['edt']; print_r($edt); # Prints Eastern Daylight Time information
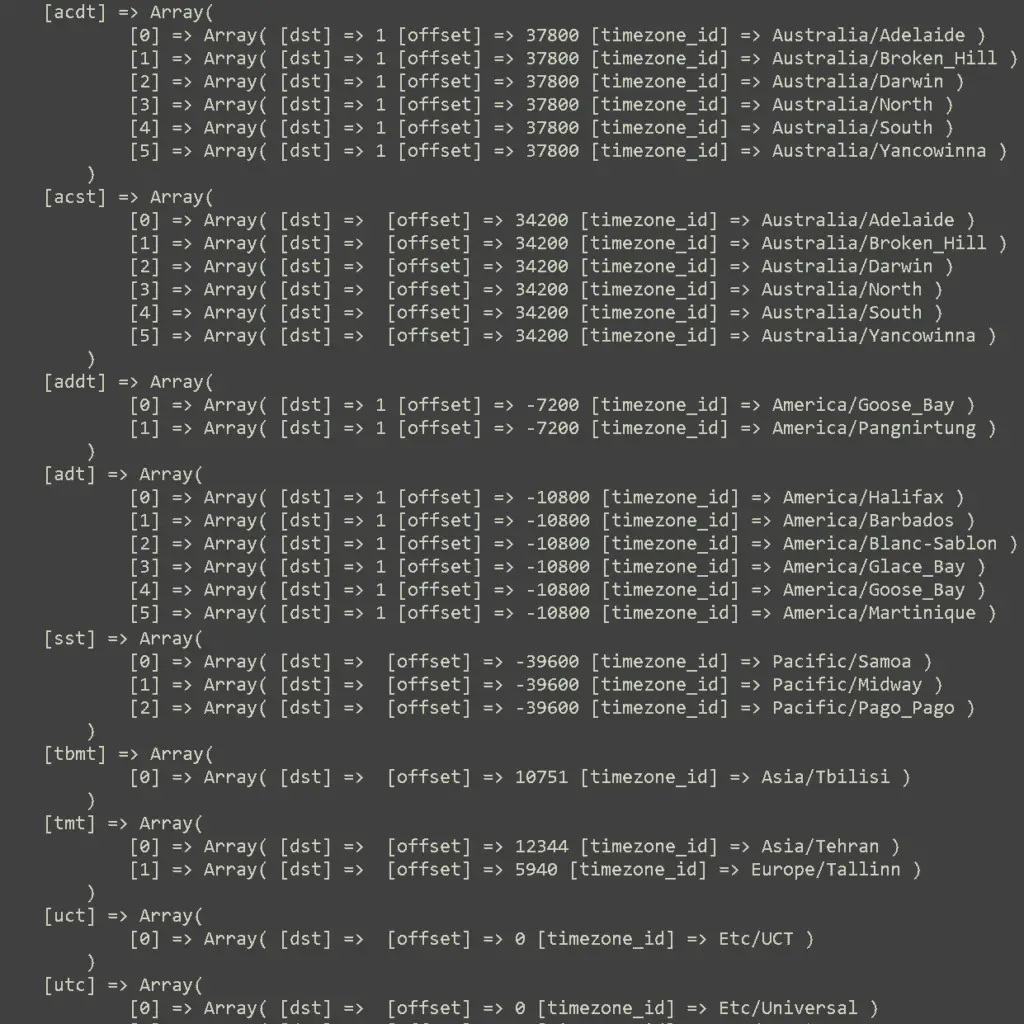
DateTimeZone::listIdentifiers
How to get all defined timezone identifiers?
The listIdentifiers()
is a static method, it returns an array of all PHP time zone identifiers.
<?php $all_identifiers = DateTimeZone::listIdentifiers(); print_r($all_identifiers); /* Prints: Array ( [0] => Africa/Abidjan [1] => Africa/Accra [2] => Africa/Addis_Ababa [3] => Africa/Algiers [4] => Africa/Asmara ... ... [422] => Pacific/Tongatapu [423] => Pacific/Wake [424] => Pacific/Wallis [425] => UTC )
Get time zone identifiers for a specific region
The listIdentifiers()
method takes two arguments:
$timezoneGroup
This argument accepts one of the DateTimeZone class constants (or a combination) listed below to return time zone identifiers for a specific region.$countryCode
The second argument accepts a two-letter (uppercase) country code to return a time zone identifier for a specific country. To get an identifier for a specific country you must provide theDateTimeZone::PER_COUNTRY
in the first argument.
List of DateTimeZone class constants:
- DateTimeZone::AFRICA
- DateTimeZone::AMERICA
- DateTimeZone::ANTARCTICA
- DateTimeZone::ARCTIC
- DateTimeZone::ASIA
- DateTimeZone::ATLANTIC
- DateTimeZone::AUSTRALIA
- DateTimeZone::EUROPE
- DateTimeZone::INDIAN
- DateTimeZone::PACIFIC
- DateTimeZone::UTC
- DateTimeZone::ALL
- DateTimeZone::ALL_WITH_BC
- DateTimeZone::PER_COUNTRY
Example: Get time zone identifiers for the Australia
<?php $i = DateTimeZone::listIdentifiers(DateTimeZone::AUSTRALIA); foreach ($i as $identifier){ echo "$identifier <br>"; } /* Prints: Australia/Adelaide Australia/Brisbane Australia/Broken_Hill Australia/Currie Australia/Darwin Australia/Eucla Australia/Hobart Australia/Lindeman Australia/Lord_Howe Australia/Melbourne Australia/Perth Australia/Sydney */
Get time zone identifier by country
The second argument of listIdentifiers()
method accepts a two-letter (uppercase) country code to return a time zone identifier for a specific country. To get an identifier for a specific country you must provide the DateTimeZone::PER_COUNTRY
in the first argument:
Example: Get time zone identifiers for USA
<?php $i = DateTimeZone::listIdentifiers(DateTimeZone::PER_COUNTRY, 'US'); foreach ($i as $identifier){ echo "$identifier <br>"; } /* Prints: America/Adak America/Anchorage America/Boise America/Chicago America/Denver America/Detroit America/Indiana/Indianapolis America/Indiana/Knox America/Indiana/Marengo America/Indiana/Petersburg America/Indiana/Tell_City America/Indiana/Vevay America/Indiana/Vincennes America/Indiana/Winamac America/Juneau America/Kentucky/Louisville America/Kentucky/Monticello America/Los_Angeles America/Menominee America/Metlakatla America/New_York America/Nome America/North_Dakota/Beulah America/North_Dakota/Center America/North_Dakota/New_Salem America/Phoenix America/Sitka America/Yakutat Pacific/Honolulu */
Example: Get time zone identifiers for China
<?php $i = DateTimeZone::listIdentifiers(DateTimeZone::PER_COUNTRY, 'CN'); foreach ($i as $identifier){ echo "$identifier <br>"; } /* Prints: Asia/Shanghai Asia/Urumqi */
The Date and Time Tutorials:
- PHP DateTime Class
- PHP DateTimeZone Class – times in different countries
- PHP DateInterval Class – adds or subtracts dates/times
- PHP DatePeriod Class – generates date or time ranges
- PHP Validating Age and Date of Birth
- Sunset, Sunrise, Transit, and Twilight
- Localizing Dates
- Localizing Dates with IntlDateFormatter Class