All the core XML extensions, for example, SimpleXML, DOM, XML Parser, XMLWriter, etc. rely on the libxml extension. This extension provides some functions to handle errors that may occur when something is wrong with the XML structure.
Example: Parsing XML Errors with libxml:
By default, PHP displays errors if it fails to parse an XML document, but it does not tell the exact location (i.e. line number, column number, etc.) of the mistake in the XML file. The following code demonstrates how you can use libxml functions to retrieve errors if PHP failed to parse an XML file:
<?php libxml_use_internal_errors(TRUE); //$xml = simplexml_load_file('quotes.xml'); $dom = new DOMDocument; $dom->load('quotes.xml'); $errorObj = libxml_get_errors(); if (!empty($errorObj)) { //var_dump($errorObj); foreach ( $errorObj as $error ) { switch ( $error->level ) { case LIBXML_ERR_FATAL: echo "Fata Error: "; break; case LIBXML_ERR_ERROR: echo "Error: "; break; case LIBXML_ERR_WARNING: echo "Warning: "; break; } echo $error->code .'<br>'. 'Message: ' . $error->message .'<br>'. 'Line: ' . $error->line .'<br>'. 'Column: ' . $error->column .'<br>'. 'File: ' . $error->file .'<hr>'; } libxml_clear_errors(); }
- The
libxml_user_internal_errors(true)
function suppress the errors. - The
libxml_get_errors
function returns an array containing the errors or returns an empty array if the XML file is parsed successfully. - Using foreach loop you can iterate over each error returned by the libxml_get_errors function.
- The
libxml_clear_errors
function clears the libxml error buffer.
The XML used in the example, we deliberately made some mistakes in the XML tags to test our code:
<?xml version="1.0"?> <quotes> <quote year="2023"> <coding>Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua...</coding> <author>Author XYZ</author> </quote> <quote year="2022"> <coding>Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua...</coding> <author>Author ABC</author> </quotee> <!-- </quote> --> </quotess> <!-- </quotes> -->
When we run the script, we receive the following output:
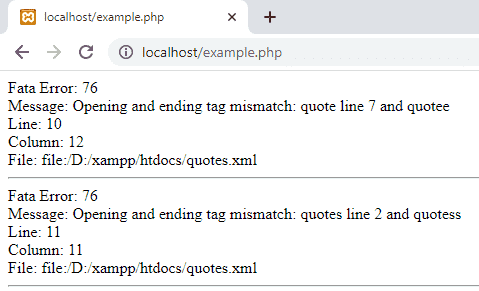
For details, visit https://php.net/manual/book.libxml.php.
Using XML: