Removing excessive whitespaces between words
Clean up the text by removing all the white space in a string, such as extra spaces, tabs, newlines, and so on. In the following code, we’ll use preg_replace
regex function to clean up the text:
<?php $string = "It is the sample text "; $newString = preg_replace('/\s+/',' ', $string); //It is the sample text
Example: Removing whitespaces but not newlines:
If you need to remove all the white space in a string except the newlines, use the /[^\S\r\n]/
regex pattern:
\S
any character that is not a whitespace character\r
carriage return\n
newline
<?php $string = 'It is the sample text. It is the sample text. It is the sample text It is the sample text.'; $newString = preg_replace('/[^\S\r\n]+/',' ', $string); // Printing original text echo '<pre>'; echo nl2br( $string ); // Printing cleaned text echo '<hr>'; echo nl2br( $newString );
The preceding code prints the following output on the web browser:
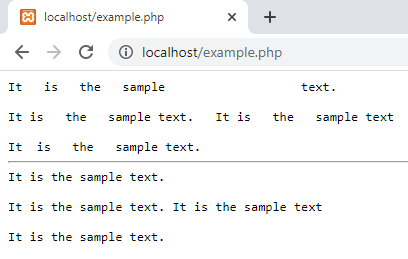
Removing spaces from the beginning and end of a string
We’ll use ltrim()
, rtrim()
, or trim()
. The ltrim()
function removes whitespace (such as newline, carriage return, space, horizontal and vertical tab, and null) from the beginning of a string, rtrim()
from the end of a string, and trim()
from both the beginning and end of a string.
Example with rtrim()
function:
$text = ' hello world '; $trimmedText = rtrim($text); echo $trimmedText;
The above code outputs hello world
, trimmed all spaces from the right side.
Example with ltrim()
function:
$text = ' hello world '; $trimmedText = ltrim($text); echo $trimmedText;
The above code outputs hello world
, trimmed all spaces from the left side.
Example with trim()
function:
$text = ' hello world '; $trimmedText = trim($text); echo $trimmedText;
The above code outputs hello world, trimming all spaces from both sides of the text.
More Regular Expressions Tutorials:
- Regular Expressions
- Matching patterns using preg_match() and preg_match_all()
- Splitting long paragraphs using preg_match_all() function
- Cleanup Text by Removing Extra Spaces (Except Newlines)
- Search and replace with preg_replace() and preg_filter()
- Search and replace with preg_replace_callback() and preg_replace_callback_array()