For example, if you want to check if a web server is online on port 80, you can use the following code. We will use the HTTP HEAD
request method which is similar to the GET
method, except that it only returns the headers and status line of the response. The HEAD
method can be useful for checking the availability, size, or modification date of a resource.
Example: Checking the status of a server
<?php $server = 'brainbell.com'; $port = '80'; $status = 'unavailable'; $fp = @fsockopen($server, $port, $errno, $errstr, 10); if ($fp) { $status = 'alive, but not responding'; // Sending HEAD request fwrite($fp, "HEAD / HTTP/1.1\r\n"); fwrite($fp, "Host: $server:$port\r\n\r\n"); if (strlen(@fread($fp, 1024)) > 0) { $status = 'alive and running'; } fclose($fp); } echo "The server is $status.";
This works well if only one server’s status must be checked. If there are several servers, this approach has some disadvantages. The call to fsockopen()
might take up to the timeout value until the next command is executed. Therefore, all servers are checked sequentially and synchronously. This just takes too long for several servers.
PHP: Check the Status of Multiple Servers Asynchronously
You can use the guzzlehttp/guzzle library to asynchronously check the status of multiple servers in PHP. This library sends asynchronous requests and handles the responses.
You can install Guzzle using Composer (https://getcomposer.org):
composer require guzzlehttp/guzzle
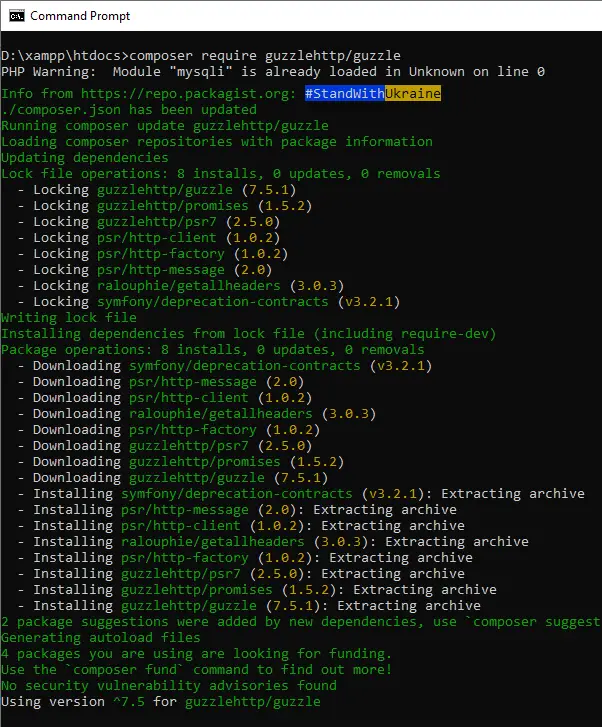
composer require guzzlehttp/guzzle
Visit: https://docs.guzzlephp.org/en/stable/quickstart.html
Then, you can create a Guzzle client and use the headAsync
method to send asynchronous requests to multiple servers. Here is a simple example of how to do it:
<?php //Autoload the Composer libraries require_once 'vendor/autoload.php'; $client = new \GuzzleHttp\Client(); // Define the servers to check $servers = ['http://brainbell.com', 'https://brainbell.net', 'https://brainbell.org']; // Create an array of promises $promises = array(); // Use headAsync for the HTTP Head request method foreach ($servers as $server) { $promises[$server] = $client->headAsync($server); } // Wait for all the promises to complete $results = \GuzzleHttp\Promise\settle($promises)->wait(); // Loop through the results and print the status code for each server foreach ($results as $server => $result) { if ($result['state'] === 'fulfilled') { // The request was successful echo '<b>'. $result['value']->getStatusCode() .' ' . $result['value']->getReasonPhrase() .'</b> ' . $server. '<br>'; } else { // The request failed echo $server . ' failed with ' . $result['reason']->getMessage() .'<br>'; } }
Communicating with Servers: