However, writing an entire site using ISAPI DLLs can be daunting. Writing ISAPI DLLs in C or C++ gives you complete control over how your site will perform and makes the site work. However, along with that control comes an equal amount of responsibility, because developing software using C or C++ presents numerous challenges.
So in delivering ASP, Microsoft provided a single IASPI DLL named ASP.DLL. ASP Web developers write their code into files tagged with the extension .asp (for example, foo.asp). ASP files often contain a mixture of static HTML and executable sections (usually written in a scripting language) that emit output at runtime. For example, the code in Listing 1-5 shows an ASP program that spits out the HelloWorld page, which contains both static HTML and text generated at runtime. (The file name is HelloWorld.asp in the accompanying examples.)
Listing 1-5
<%@ Language="javascript" %> <html><body><form> <h3>Hello world!!! This is an ASP page.</h3> <% Response.Write("This content was generated ");%> <% Response.Write("as part of an execution block");%> </form> </body></html>
The code shown in Listing 1-5 renders the following page. IIS watched port 80 for requests. When a request for the file Helloworld.asp came through, IIS saw the .asp file extension and asked ASP.DLL to handle the request (that's how the file mapping was set up). ASP.DLL simply rendered the static HTML as the string "Hello world!!! This is an ASP page." Then when ASP.DLL encountered the funny-looking execution tags (<% and %>), it executed those blocks by running them through a JavaScript parser. Figure 1-2 shows how the page renders in Internet Explorer.
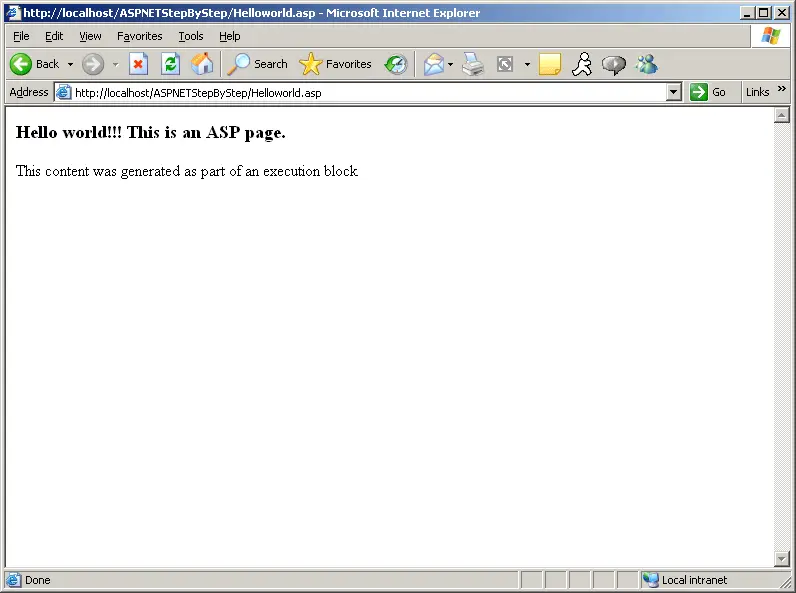
Figure 1-2 The results of a request made to the ASP program from Listing 1-5.
This tutorial is about developing ASP.NET software, so we'll focus most of the attention there. However, before leaving the topic of classic ASP, Listing 1-6 shows the SelectFeature.htm page rewritten as a classic ASP page. Looking at this simple ASP application presents some of the core issues in Web development and illustrates why Microsoft rewrote its Web server technology as ASP.NET. (The accompanying file name is SelectFeature.asp.)
Listing 1-6
<%@ Language="javascript" %> <html><body><form> <h2>HelloWorld<h2> <h3>What's your favorite .NET feature?</h3> <select name='Feature'> <option> Type-Safety</option> <option> Garbage collection</option> <option> Multiple syntaxes</option> <option> Code Access Security</option> <option> Simpler threading</option> <option> Versioning purgatory</option> </select> </br> <input type=submit name="Submit" value="Submit"></input> <p> Hi, you selected <%=Request("Feature") %> </p> </form> </body></html>
Much of the text in SelectFeature.asp looks very similar to SelectFeature.htm, doesn't it? The differences lie mainly in the first line (that now specifies a syntax for executable blocks) and the executable block marked by <% and %>. The rest of the static HTML renders a selection control within a form.
Take note of the executable blocks and how the blocks use the Response object (managed by the ASP infrastructure) to push text out to the browser. The executable block examines the Feature control (specified by the <select> tag) and prints out the value selected by the user.
Figure 1-3 shows how SelectFeature.asp renders in Internet Explorer.
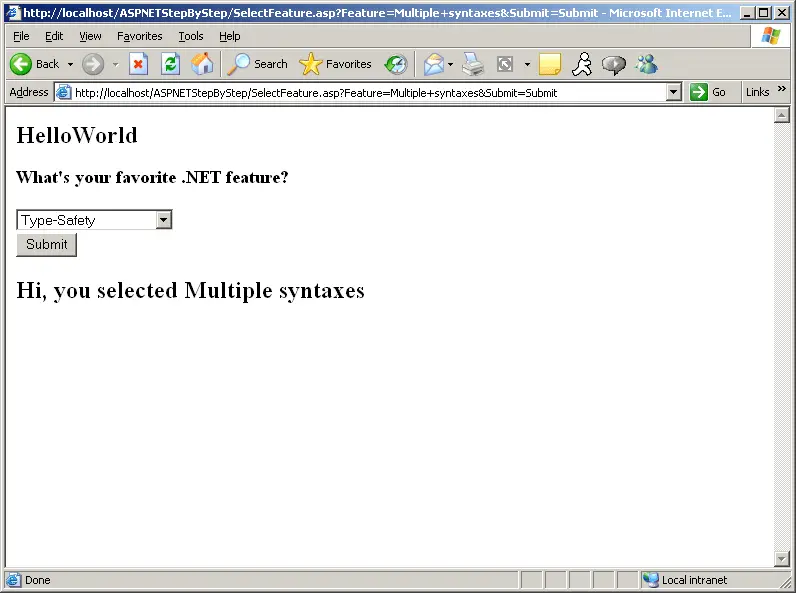
Figure 1-3 The code from Listing 1-6 as viewed using Internet Explorer.
The screen in Figure 1-3 may look a bit odd because the drop-down list box shows "Type-Safety" while the rendered text shows "Multiple syntaxes." Without doing anything extra, the drop-down list box will always re-render with the first element as the selected element. We'll see how ASP.NET fixes this later when we look at server-side controls. That's enough background information to help you understand the core issues associated with developing Web applications.