Revisiting the iTunes XML Music Library
You're going to create an XSLT stylesheet that transforms your iTunes XML library document into an HTML document that is suitable for viewing in a web browser. But before you get into the code for the stylesheet, let's take one more look at the XML code for a library file. Listing 13.2 contains partial code for an iTunes library file where the code for a music track is visible.
Listing 13.2. A Partial Listing of an iTunes Music Library.xml
Music Library Document
1: <?xml version="1.0" encoding="UTF-8"?> 2: <!DOCTYPE plist PUBLIC "-//Apple Computer//DTD PLIST 1.0//EN" "http://www.apple.com/DTDs/ 3: PropertyList-: 1.0.dtd"> 4: <?xml-stylesheet href="itunesview.xsl" type="text/xsl"?> 5: <plist version="1.0"> 6: <dict> 7: <key>Major Version</key><integer>1</integer> 8: <key>Minor Version</key><integer>1</integer> 9: <key>Application Version</key><string>4.9</string> 10: <key>Music Folder</key><string>file://localhost/C:/Documents%20and%20Settings/ 11: Test%20Name/My%20Documents/My%20Music/iTunes/iTunes%20Music/ 12: </string> 13: <key>Library Persistent ID</key><string>45B7F87C7466C64A</string> 14: <key>Tracks</key> 15: <dict> 16: ... 17: <key>37</key> 18: <dict> 19: <key>Track ID</key><integer>37</integer> 20: <key>Name</key><string>Thinking Of You</string> 21: <key>Artist</key><string>Lenny Kravitz</string> 22: <key>Composer</key><string>Lenny Kravitz/Lysa Trenier</string> 23: <key>Album</key><string>5</string> 24: <key>Genre</key><string>Pop/Funk</string> 25: <key>Kind</key><string>MPEG audio file</string> 26: <key>Size</key><integer>6141310</integer> 27: <key>Total Time</key><integer>383764</integer> 28: <key>Track Number</key><integer>32</integer> 29: <key>Year</key><integer>1998</integer> 30: <key>Date Modified</key><date>2005-06-08T20:04:06Z</date> 31: <key>Date Added</key><date>2004-05-06T04:29:57Z</date> 32: <key>Bit Rate</key><integer>128</integer> 33: <key>Sample Rate</key><integer>44100</integer> 34: <key>Comments</key><string>By ScazzI</string> 35: <key>Play Count</key><integer>6</integer> 36: <key>Play Date</key><integer>-1088231274</integer> 37: <key>Play Date UTC</key><date>2005-08-13T05:00:22Z</date> 38: <key>Track Type</key><string>File</string> 39: <key>Location</key><string>file://localhost/C:/ 40: Documents%20and%20Settings/Test%20Name/My%20Documents/ 41: My%20Music/Masheed/Lenny%20Kravitz%20-%20Thinking%20Of%20You.mp3/ 42: </string> 43: <key>File Folder Count</key><integer>-1</integer> 44: <key>Library Folder Count</key><integer>-1</integer> 45: </dict> 46: ... 47: </dict> 48: </plist>
This XML-coded iTunes music track contains all the information you need to crank out an XSLT stylesheet that rips out the important information and transforms it into something pretty that can be viewed in a web browser. Just in case you aren't seeing it right off, lines 18 through 45 contain the key-value pairings for the song "Thinking Of You" by Lenny Kravitz.
Because stylesheets aren't magically associated with XML documents automatically, you must make a small addition to your XML music library document in order to wire it to the XSLT stylesheet that you're about to see. This line of code is already present in the XML library document shown in Listing 13.2. Just check out line 4 to see how the familiar xml-stylesheet
directive is used to link the stylesheet to the XML document. Make sure to add this line of code to your own XML library document or the stylesheet will have no effect.
Inside the iTunes Viewer XSLT Stylesheet
The stylesheet that transforms the iTunes music library document is really only interested in three pieces of information in the document: the track name, artist, and album. This information is relatively easy to find within the document because the <key>
tag contains the text Name
, Artist
, and Album
for each piece of information, respectively. Therefore, the job of the stylesheet is to look through the XML code for tags whose content is equal to Name
, Artist
, or Album
, and then grab the contents of the adjacent "value" tag (<string>
in this case).
Listing 13.3 contains the complete code for the itunesview.xsl_XSLT
stylesheet, which transforms the tracks in an iTunes music library document into a nicely formatted list complete with links back to the iTunes music store.
Listing 13.3. The itunesview.xsl
XSLT Stylesheet Transforms an iTunes Music Library into a Cleanly Formatted HTML Web Page
1: <?xml version="1.0"?> 2: <xsl:stylesheet version="1.0" xmlns:xsl="http://www.w3.org/1999/XSL/Transform"> 3: <xsl:template match="/"> 4: <html><head><title>My iTunes Songs</title></head> 5: <style> 6: <xsl:comment> 7: h1 { 8: text-align:center; 9: font-family:arial; 10: font-size:18pt; 11: color:#669966; 12: } 13: table { 14: border-style:none; 15: padding:0px; 16: margin:0px; 17: text-align:left; 18: } 19: th { 20: font-family:arial; 21: font-size:14pt; 22: background-color:#669966; 23: color:#FFFFFF; 24: } 25: 26: td { 27: font-family:arial; 28: font-size:11pt; 29: color:#669966; 30: } 31: 32: a:link { 33: text-decoration:none; 34: color:#669966; 35: } 36: 37: a:hover { 38: font-weight:bold; 39: text-decoration:none; 40: color:#66BB66; 41: } 42: 43: a:visited { 44: text-decoration:none; 45: color:#333333; 46: } 47: </xsl:comment> 48: </style> 49: 50: <body> 51: <h1>My iTunes Songs</h1> 52: <table style="width:100%"> 53: <tr style="font-weight:bold"> 54: <th style="width:40%">Name</th> 55: <th style="width:20%">Artist</th> 56: <th style="width:40%">Album</th> 57: </tr> 58: <xsl:call-template name="main" /> 59: </table> 60: </body> 61: </html> 62: </xsl:template> 63: 64: <xsl:template name="main"> 65: <xsl:for-each select="/*/*/dict[1]/dict"> 66: <xsl:element name="tr"> 67: <xsl:call-template name="song" /> 68: </xsl:element> 69: </xsl:for-each> 70: </xsl:template> 71: 72: <xsl:template name="song"> 73: <tr> 74: <xsl:if test="position() mod 2 != 1"> 75: <xsl:attribute name="style">background-color:#EEEEEE 76: </xsl:attribute> 77: </xsl:if> 78: 79: <td style="height=20px"> 80: <xsl:element name="a"> 81: <xsl:attribute name="href"> 82: itms://phobos.apple.com/WebObjects/MZSearch.woa/wa/ 83: advancedSearchResults?songTerm=<xsl:value-of select= 84: "child::*[preceding-sibling::* = 'Name']" />&artistTerm= 85: <xsl:value-of select="child::*[preceding-sibling::* = 86: 'Artist']" />&albumTerm=<xsl:value-of select= 87: "child::*[preceding-sibling::* = 'Album']" /> 88: </xsl:attribute> 89: <img src="itunes.gif" alt="iTunes" style="border:none" /> 90: <xsl:text> </xsl:text><xsl:value-of select= 91: "child::*[preceding-sibling::* = 'Name']" /> 92: </xsl:element> 93: </td> 94: 95: <td> 96: <xsl:element name="a"> 97: <xsl:attribute name="href"> 98: itms://phobos.apple.com/WebObjects/MZSearch.woa/wa/ 99: advancedSearchResults?artistTerm=<xsl:value-of select= 100: "child::*[preceding-sibling::* = 'Artist']" /> 101: </xsl:attribute> 102: <xsl:value-of select="child::*[preceding-sibling::* = 103: 'Artist']" /> 104: </xsl:element> 105: </td> 106: 107: <td> 108: <xsl:element name="a"> 109: <xsl:attribute name="href"> 110: itms://phobos.apple.com/WebObjects/MZSearch.woa/wa/ 111: advancedSearchResults?artistTerm=<xsl:value-of select= 112: "child::*[preceding-sibling::* = 'Artist']" />&albumTerm= 113: <xsl:value-of select="child::*[preceding-sibling::* = 114: 'Album']" /> 115: </xsl:attribute> 116: <xsl:value-of select="child::*[preceding-sibling::* = 117: 'Album']" /> 118: </xsl:element> 119: </td> 120: </tr> 121: </xsl:template> 122: </xsl:stylesheet>
I realize this is a pretty hefty listing but don't let it intimidate you. If you take a closer look at the code, you'll realize that almost the first half of it is an internal CSS stylesheet aimed at making the resulting HTML document more visually appealing. In other words, there is nothing critical in lines 5 through 48 that would prevent the XSLT stylesheet from working if you deleted those lines. However, the internal CSS stylesheet is still useful in that it formats and styles the output so that it is easier to view.
The real meat of the XSLT stylesheet begins on line 72 where the song template begins. This template is initiated from line 67 within the main template upon finding nested <dict>
tags. If you refer back to Listing 13.2 (lines 15 through 18), you'll notice that songs in the music library are coded within <dict>
tags (one for each song) that are nested within a higher level <dict>
tag. The main template in the stylesheet uses this relationship as the basis for determining if it has encountered song data. If so, the song template is called to further process the song data.
The song template comprises the remainder of the stylesheet. It starts off by performing a sneaky little trick to draw an alternating background color for each song (lines 74 to 77). This gives the resulting page a horizontally striped appearance that helps you distinguish each song from the next. To alternate the background color for each song, the position()
function is called to determine the position of the current node. The specific value that this function returns isn't really important so long as it serves as a counter as you progress through the songs. The mod
operator that is applied to the return value of the position()
function results in a different background color being applied for evenly numbered rows of song data.
After the background color trick, the song template moves on to laying out the cell data for the three table cells (song, artist, and album) that represent a song row in the HTML table. The first cell is the song cell, and it consists of the song name coded as an anchor link that links to the song in the iTunes music store. Lines 80 through 92 reveal how the <a>
tag is coded for the song link, including the dynamically assembled HRef
attribute (lines 81 through 88), an iTunes logo image (line 89), and the actual song name itself (lines 90 and 91). The remaining code in the song template carries out a similar task for the artist and album cells within the table.
Viewing the End Result in a Browser
You're finally ready to see the end result of all your hard work. Just open your music library XML document in a web browser to view it transformed as an HTML web page. Figure 13.4 shows a sample iTunes library as viewed in Internet Explorer.
Figure 13.4. This iTunes music library has been successfully transformed thanks to the itunesview.xsl
XSLT stylesheet.
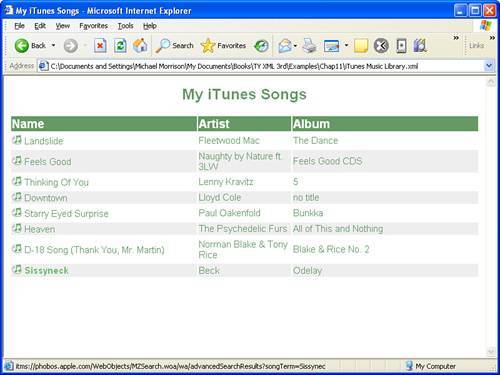
If for some reason your iTunes music library isn't styled when you open it in a web browser, check to make sure that you've added the line of code that links the itunesview.xsl
stylesheet to the XML document (see line 4 of Listing 13.2).
As the figure reveals, all of the tracks in the iTunes XML library document are transformed into a cleanly formatted web page that is easy to read. Perhaps even more interesting is how the songs, artists, and albums all serve as links to relevant areas of the iTunes music store. By clicking any of these links you are led directly to the song, artist, or album in the music store. Figure 13.5 shows the iTunes application after clicking the song Sissyneck in Figure 13.4.
Figure 13.5. Clicking a song on the transformed web page takes you to the song in the iTunes music store.
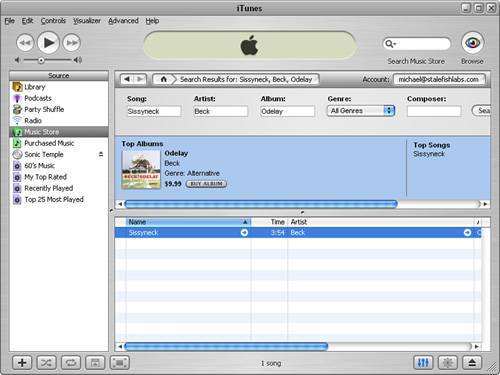
As promised, clicking the song in the music library web page leads straight to the song in the music library within the iTunes application. So, if this page is published on the Web, anyone who visits it and clicks a link will be led to the appropriate search result within her own iTunes desktop application, which is where the music store is located.