PHP.net listed the following web services in its documentation:
- OAuth
It is an authorization protocol built on top of HTTP which allows applications to securely access data without having to store usernames and passwords. - SOAP
It is an RPC-style service, PHP has a built-in extension for both client and server. - Yar (Yet Another RPC Framework)
This PECL extension is not bundled with PHP. - XML-RPC
This extension is experimental and not compiled by default, you need to add--with-xmlrpc
to your configure line when you compile PHP.
YAR – Yet Another RPC Framework
Enable Yar RPC on Windows (Xampp)
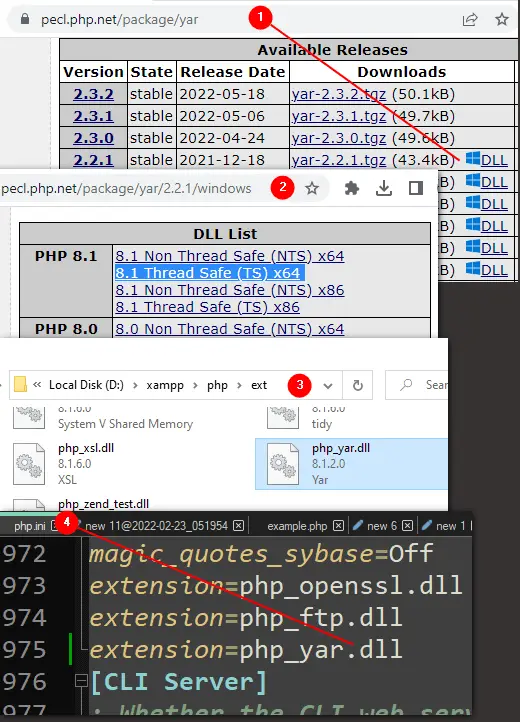
- Visit https://pecl.php.net/package/yar
- Choose the latest DLL download link (2.2.1 at the time of writing this tutorial)
- On the next page, choose a download link according to your PHP version. For example, choose “8.1 Thread Safe (TS) x64” if your Windows is 64-bit and installed the PHP version is 8.1.
- Extract the downloaded zip archive.
- Copy
php_yar.dll
file and paste it into thexampp/php/ext
folder. - Open
xampp/php/php.ini
file and add the following line
extension=php_yar.dll, and save the changes. - Restart the Apache web server
For more information, visit https://php.net/manual/install.pecl.php.
Creating a Web Service with YAR
To provide Web Service with Yar, you have to instantiate a class, the preceding code creates a RPC server for a simple Add, Subtract, and Multiply Web Service:
<?php /* this page can be accessed by http://locahost/yar_server.php */ class Mathematics { /** * BrainBell.com * Add two numbers * @param interge * @return interge */ public function sum($a, $b) { return $this->_sum($a, $b); } /** * BrainBell.com * Subtract */ public function subtract($a, $b) { return $a - $b; } /** * BrainBell.com * Multiply */ public function multiply($a, $b) { return $a * $b; } /** * Protected methods will not be exposed * @param interge * @return interge */ protected function _sum($a, $b) { return $a + $b; } } $object = new Mathematics(); $server = new Yar_Server($object); $server->handle();
To set up an HTTP RPC Server:
- $object = new Mathematics();
Initiate the class you want to register as the web service. - $server = new Yar_Server($object);
Set up an RPC Server, all the public methods of$object
will be registered as an RPC service. - $server->handle();
Starts an RPC server, and is ready to accept RPC requests.
When you access the server URL in the browser. The above code will output something like this:
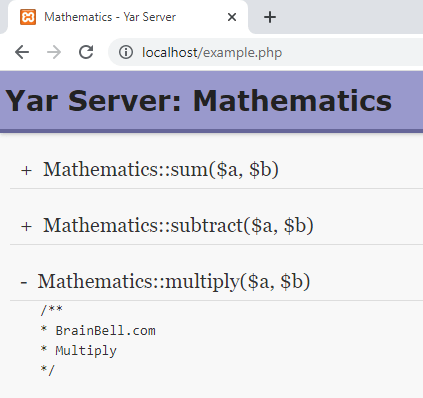
Consuming a Web Service with YAR
Querying the Web Service is rather easy. Just instantiate the yar_client
class (providing the Yar Server URL), then call the object methods with all the parameters. You receive the result of the Web Service call in return or an error message:
<?php /* this page can be accessed by http://locahost/yar_client.php */ $client = new yar_client("http://localhost/example.php"); $sum = $client->sum(23, 19); // Mathematics::sum var_dump($sum); //int(42) // OR use call method $sub = $client->call('subtract', [42, 18]); // Calls Mathematics::subtract var_dump($sub); //int(24)
Async Calls to RPC Server
Use the Yar_Concurrent_Client class for async calls to the RPC server:
<?php /* this page can be accessed by http://locahost/yar_client_async.php */ function cbFunc($result, $info) { echo $info['method'] , ": ", $result , '<br>'; } $url = 'http://localhost/example.php'; $sum = array(24, 26); $sub = array(26, 24); $mul = array(10, 10); Yar_Concurrent_Client::call($url, 'sum', $sum, "cbFunc"); Yar_Concurrent_Client::call($url, 'subtract', $sub, "cbFunc"); Yar_Concurrent_Client::call($url, 'multiply', $mul, "cbFunc"); /* sent all request and wait for response */ Yar_Concurrent_Client::loop();
For more information, visit https://php.net/manual/class.yar-concurrent-client.php.
Communicating with Servers: