To use XSL Transformations (XSLT) with PHP, you have to enable extension=xsl
in php.ini
.
The following code transforms the quotes.xml to HTML by applying the stylesheet given by the XSLTProcessor::importStylesheet()
method:
<?php $xml = new DOMDocument(); $xml->load('quotes.xml'); $xsl = new DOMDocument(); $xsl->load('style.xsl'); $xslt = new XsltProcessor(); $xslt->importStylesheet($xsl); $result = $xslt->transformToDoc($xml); echo $result->saveXML();
Doing the transformation is a number of five easy steps:
- Load the XML
- Load the XSL
- Instantiate an XsltProcessor object
- Import XSL with importStylesheet()
- Execute the transformation with transformToDoc() and print the result.
The preceding coding contains the code for these steps. The file style.xsl
contains markup that transforms the quotes.xml
into the well-known HTML tags.
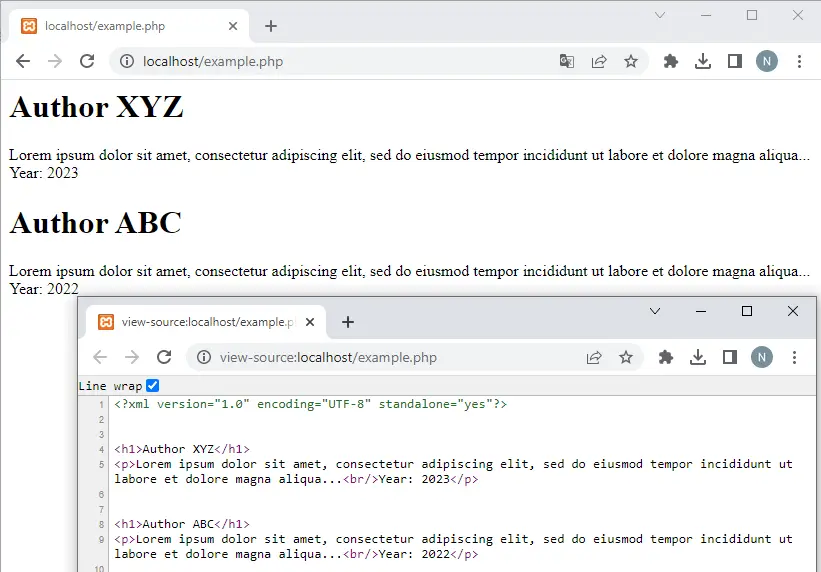
Note: The XSLTProcessor::transformToDoc
returns a DOMDocument
object, you can use the XSLTProcessor::transformToXml
for a string value:
<?php $xml = new DOMDocument(); $xml->load('quotes.xml'); $xsl = new DOMDocument(); $xsl->load('style.xsl'); $xslt = new XsltProcessor(); $xslt->importStylesheet($xsl); echo $xslt->transformToXML($xml);
The above code returns the following output (source code):
<h1>Author XYZ</h1> <p>Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua... <br>Year: 2023</p> <h1>Author ABC</h1> <p>Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua... <br>Year: 2022</p>
The quotes.xml file:
<?xml version="1.0"?> <quotes> <quote year="2023"> <coding>Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua...</coding> <author>Author XYZ</author> </quote> <quote year="2022"> <coding>Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua...</coding> <author>Author ABC</author> </quote> </quotes>
The style.xsl file:
<?xml version="1.0" encoding="UTF-8"?> <xsl:stylesheet version="1.0" xmlns:xsl="http://www.w3.org/1999/XSL/Transform"> <xsl:output method="html" encoding="UTF-8" indent="no"/> <xsl:template match="quote"> <h1><xsl:value-of select="author"/></h1> <p><xsl:value-of select="coding"/> <br />Year: <xsl:value-of select="@year"/> </p> </xsl:template> </xsl:stylesheet>
To learn about the XSLT and XML visit Transforming XML with XSLT.
Using XML: