A paragraph is a series of sentences, when breaking a large text or paragraph we have to take care that a sentence should not be split into two paragraphs.
We’ll create a custom PHP function that accepts two parameters:
$text
: the String to be processed.$minLength
: the minimum number of characters in a paragraph.$needle
: default is . (dot).
This function will return an array filled with short paragraphs.
The breakLongText
function:
<?php function breakText($text, $minLength = 200, $needle='.') { $delimiter = preg_quote($needle); $match = preg_match_all("/.*?$delimiter/",$text, $matches); if ($match == 0) return array($text); $sentences = current($matches); $paras = array(); $tmp = ''; foreach ($sentences as $sentence) { $tmp .= $sentence; if (strlen($tmp) > $minLength){ $paras[] = $tmp; $tmp = ''; } } if ($tmp != '') $paras[] = $tmp; return $paras; } $text = 'Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.'; $ps = breakText($text,300); foreach ($ps as $s) echo "<p>$s</p>";
The preceding code prints the following output on the browser window:
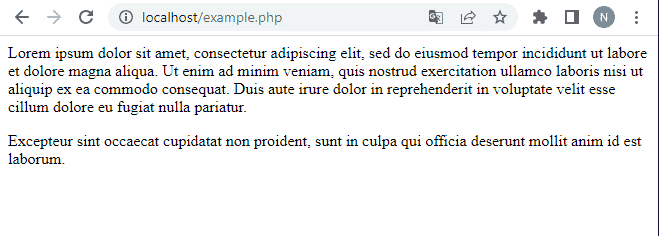
This function will always return an array even the if the text is not processed i.e. for already short text or $needle is not found in the text.
More Regular Expressions Tutorials:
- Regular Expressions
- Matching patterns using preg_match() and preg_match_all()
- Splitting long paragraphs using preg_match_all() function
- Cleanup Text by Removing Extra Spaces (Except Newlines)
- Search and replace with preg_replace() and preg_filter()
- Search and replace with preg_replace_callback() and preg_replace_callback_array()