After the form has been submitted, the data must be stored somewhere i.e. in a database, in a file, or sent via email. When a website contains several similar forms (for example, forms requiring the user to provide his name and contact information), it is a good idea to save the data after the user fills it in. Because HTTP is a stateless protocol, you have to use cookies.
Saving Data into a Cookie
<?php function saveFormData($post) { $keys = implode('-;-', array_keys($post) ); $vals = implode('-;-', array_values($post) ); $data = "$keys:;:$vals"; $expire = time() + 86400 * 30; //30 days setcookie('data',$data, $expire, '/', 'brainbell.com'); }
Because user agents only have to save 20 cookies per domain, it’s a good idea to store the form information in one cookie, in the form of an array. But, only string values are allowed in cookies; this is why you have to convert the $_POST
array into a string.
The function getCookieData()
retrieves the existing data from the cookie and converts it into an array and returns it (or returns false if data is unavailable):
<?php function getCookieData() { if (! isset($_COOKIE['data']) ) return false; $data = explode(':;:', $_COOKIE['data']); $keys = explode('-;-', $data[0]); $vals = explode('-;-', $data[1]); return array_combine($keys, $vals); }
The only thing left to do is to write the required form data into this array. You can specifically submit only certain values or the complete array $_GET
or $_POST
, as shown in the following code.
Saving (and retrieving) form data in a cookie:
<?php if ($_SERVER['REQUEST_METHOD'] == 'POST') { saveFormData( $_POST ); } else { printForm(); } function saveFormData($post) { $keys = implode('-;-', array_keys($post) ); $vals = implode('-;-', array_values($post) ); $data = "$keys:;:$vals"; $expire = time() + 86400 * 30; //30 days setcookie('data',$data, $expire, '/'); echo '<h1>Form Saved in a Cookie</h1> <p><a href="example.php">Go back</a></p>'; } function getFormData() { if (! isset($_COOKIE['data']) ) return false; $data = explode(':;:', $_COOKIE['data']); $keys = explode('-;-', $data[0]); $vals = explode('-;-', $data[1]); return array_combine($keys, $vals); } function printForm(){ $data = getFormData(); if ($data === false){ echo '<p><b>You\'ve previously not submitted the form.</b></p>'; } else{ echo '<p><b>Your previously submitted values:</b></p>'; echo '<pre>'; print_r($data); echo '</pre>'; } ?> <form method="post" action=""> <div>Name: <input type="text" name="name"></div> <div>Email: <input type="email" name="email"></div> <input type="submit" name="submit" value="Submit"> </form> <?php }
Figure shows the resulting cookie:
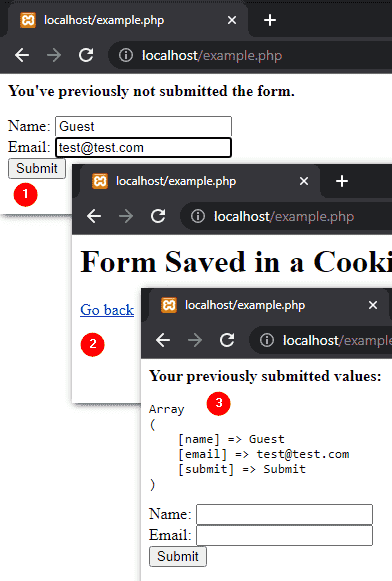
Processing Forms in PHP: