An array is an ordered set of variables, in which each variable is called an element. There are two types of arrays: numbered and associative. The first type of array uses numerical keys, whereas the latter type can also use strings as keys.
Creating Arrays
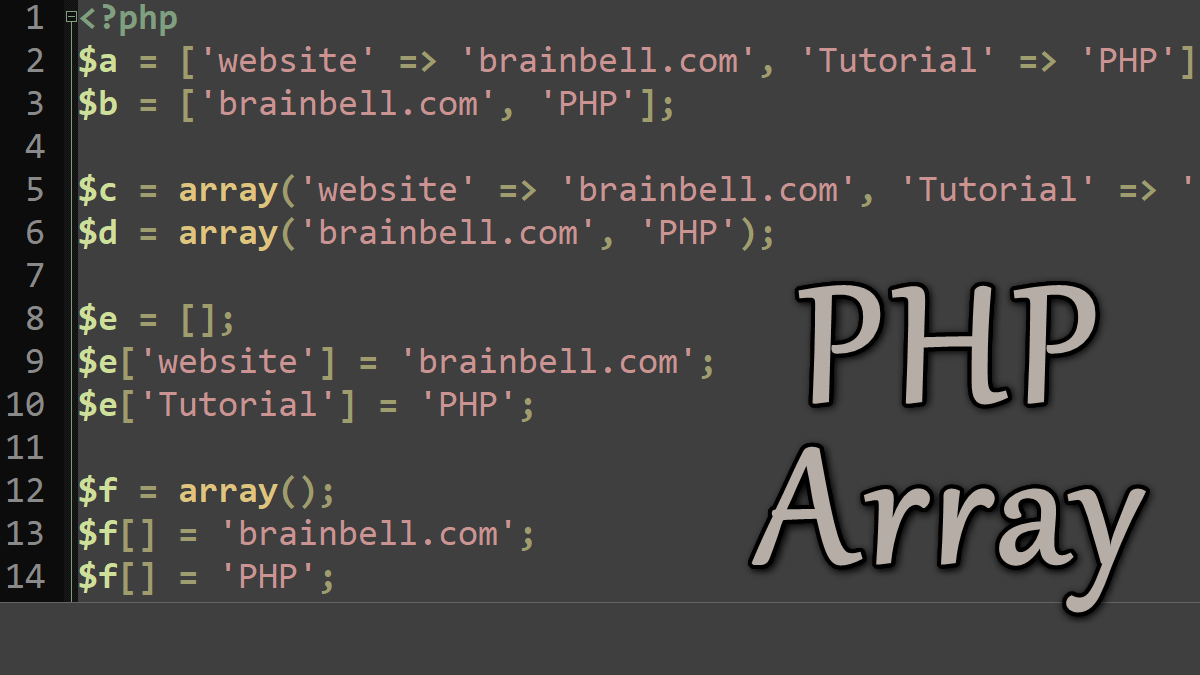