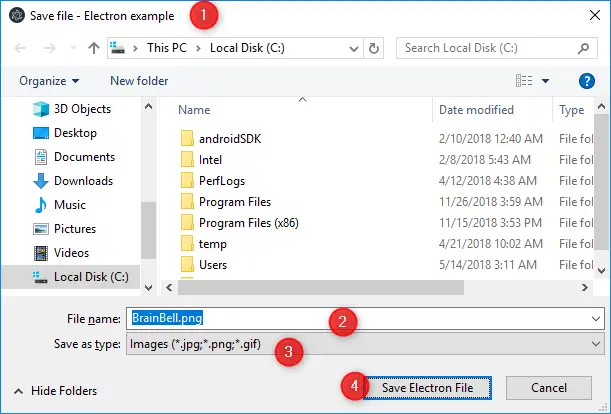
//Syntax dialog.showSaveDialog([browserWindow, ]options[, callback]) //renderer.js - renderer process example const {remote} = require('electron'), dialog = remote.dialog, WIN = remote.getCurrentWindow(); let options = { //Placeholder 1 title: "Save file - Electron example", //Placeholder 2 defaultPath : "C:\\BrainBell.png", //Placeholder 4 buttonLabel : "Save Electron File", //Placeholder 3 filters :[ {name: 'Images', extensions: ['jpg', 'png', 'gif']}, {name: 'Movies', extensions: ['mkv', 'avi', 'mp4']}, {name: 'Custom File Type', extensions: ['as']}, {name: 'All Files', extensions: ['*']} ] } //Synchronous let filename = dialog.showSaveDialog(WIN, options) console.log(filename) //Or asynchronous - using callback dialog.showSaveDialog(WIN, options, (filename) => { console.log(filename) })
You can see in above example dialog.showSaveDialog()
takes three arguments. The first is a reference to a BrowserWindow
, which is used to display the dialog box as a modal (which enables the dialog box to stay on top of its parent window). The second argument is an options
object that allows you to pass keys and values to configure the dialog box itself. The third argument is callback, if a callback is passed, the API call will be asynchronous and the result will be passed via callback(filenames)
.
The options
object works with the following options:
title
:
Sets the title of the dialog box.defaultPath
:
Sets the default directory for the Save dialog box.buttonLabel
:
Allows you to set custom text for the Save button.filters
:
Sets what files are enabled to select to overwrite.message
:
Message to display above text fields (macOS only).nameFieldLabel
:
Custom label for the text displayed in front of the filename text field (macOS only).showsTagField
:
Show the tags input box (macOS only).securityScopedBookmarks
:
Create a security scoped bookmark when packaged for the Mac App Store.
Show Error Message Dialog
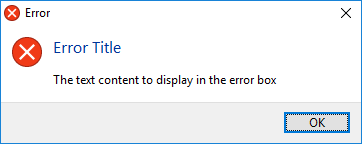
Although the dialog.showMessageBox()
does have an error type but dialog.showErrorBox(title, content)
can be called safely before the ready
event the app
module emits, it is usually used to report errors in early stage of startup. This method takes in two parameters:
title
: The content’s title to display in the dialog boxcontent
: The text content to display in the error box
//main.js - main process const {dialog} = require('electron'), title = 'Error Title', content = 'The text content to display in the error box'; dialog.showErrorBox(title, content)