Get information about characters used in a string
<?php //Syntax count_chars(string $string, int $mode = 0): array|string
$string
: the input string that you want to examine.$mode
(optional): an integer value from 0 to 4, default value is0
.
The count_chars()
function returns information about the characters found in a string. For example, you can get unique characters and byte values of each character in the given string based on the $mode
value:
0
– returns an array with the byte value (0-255) as key and the frequency of every byte as value (even if the frequency is zero).
See examples: using the mode zero and how many times a character appeared in a given string.1
– same as 0, return an array but only byte values with a frequency greater than zero.
See mode value 1 example.2
– same as 0, return an array but only byte values with a frequency equal to zero.3
– return a string containing all unique characters.
See, how to get unique characters in a given string.4
– return a string containing all unused characters.
Example: count_chars with mode value is 0
(default)
<?php $text = count_chars('BrainBell.com', 0); print_r($text);
The above example prints the returned array containing all used and unused bytes in the string. In the following figure, we highlighted the used elements in red to distinguish them from unused elements. From the following output, the element “[46]=>1
“, 46 is the byte-value and 1
is frequency, which means the byte-value 46 (.
) appeared only once in the string. You can use the chr() function to convert a byte value into its corresponding character.
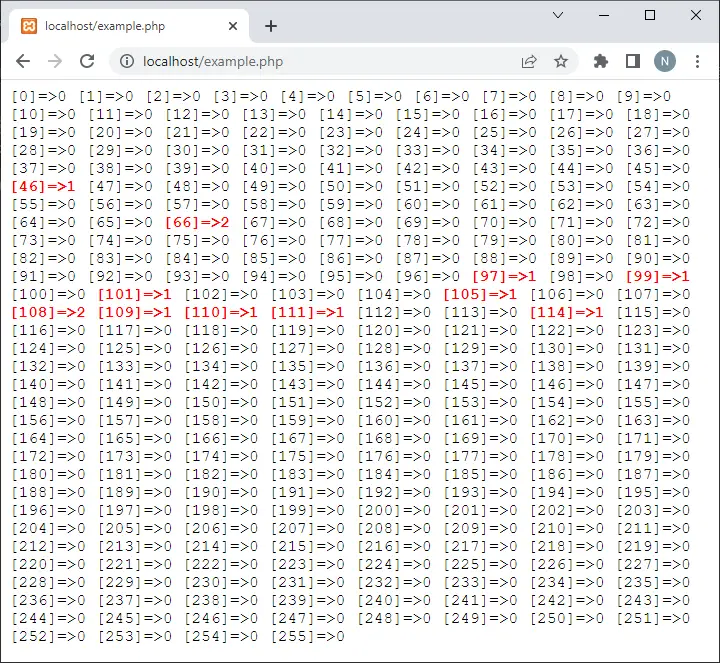
Example: get byte values and number of occurrences of each character in a string
If you assign value 1
to $mode
parameter, the count_chars()
function will returns an array with the byte value as its keys and the number of occurrences of every byte (if not zero) as its values, see example:
<?php $a = count_chars('BrainBell.com', 1); print_r($a); /*Prints: Array ( [46] => 1 [66] => 2 [97] => 1 [99] => 1 [101] => 1 [105] => 1 [108] => 2 [109] => 1 [110] => 1 [111] => 1 [114] => 1 ) */
Example: how many times a character appeared in a given string
The following example displays how many times a character appeared in the string in human-readable form:
<?php $a = count_chars('BrainBell.com', 1); foreach($a as $k=>$v) echo chr($k)." appears $v times<br>\n"; /*prints: . appears 1 times B appears 2 times a appears 1 times c appears 1 times e appears 1 times i appears 1 times l appears 2 times m appears 1 times n appears 1 times o appears 1 times r appears 1 times */
Example: get unique characters in a given string
If you assign value 3
to $mode
parameter, the count_chars()
function will return a string containing all unique characters in the given string. See example:
<?php echo count_chars('BrainBell.com', 3); #Prints: .Baceilmnor
str_word_count()
Words are defined as a string of alphabetical characters, depending on the locale settings, and may contain but not start with -
and '
.
<?php //Syntax str_word_count(string $string, int $format = 0, ?string $characters = null): array|int
The str_word_count() function has three parameters:
$string
: the string to examine.$format
(optional): an integer value 0, 1 or 2, default is 0.$characters
(optional): additional characters which will be considered as ‘word’.
By default, the str_word_count()
function returns the total number of words found in a string. You can change the default behavior by defining the $format
parameter:
0
– (default) returns total number of words.1
– returns an array containing all the words found inside the string.2
– returns an associative array, where the key is the numeric position of the word in the string and the value is the word itself.
Example: Count words in a given string
The str_word_count()
function provides an easy way to count the number of words in a string. See example:
<?php echo str_word_count('BrainBell.com BrainBell.com'); //Prints: 4
Example: Converting string to array by word
<?php $array = str_word_count('BrainBell.com BrainBell.com', 1); print_r($array); /*Prints: Array ( [0] => BrainBell [1] => com [2] => BrainBell [3] => com )*/
Example: Get the frequency of all words in a given string
Use the str_word_count()
and array_count_values()
functions to determine the frequency in which each word appears in an array.
<?php $a = str_word_count('My website: BrainBell.com BrainBell.com', 1); $b = array_count_values($a); print_r($b); /*Prints: Array ( [My] => 1 [website] => 1 [BrainBell] => 2 [com] => 2 )*/
Example: Find the position of each word in a given string
Define $format
value 2
to get the numeric position of each word.
<?php $a = str_word_count('My website: BrainBell.com BrainBell.com', 2); print_r($a); /*Prints: Array ( [0] => My [3] => website [12] => BrainBell [22] => com [26] => BrainBell [36] => com )*/
Follow this tutorial if you want to find positions of a substring in a string.
Example: add characters that will be accepted as part of a word
We know that words are alphabetical characters and may contain but not start with -
and '
. So, if a word contains a number or a non-alphabet character, that single word will breaks into two words, in the following example the string breaks into four words:
<?php $str = 'H3llö Wörld'; echo str_word_count($str); //4 $a = str_word_count($str, 1); print_r($a); /* [0] => H [1] => ll [2] => W [3] => rld */
You can use the optional third argument, $characters
, that allows you to add characters that will be accepted as part of a word, such as foreign accent marks, numbers, etc.
<?php $str = 'H3llö Wörld'; echo str_word_count($str, 0, '3ö'); //2 $a = str_word_count($str, 1, '3ö'); print_r($a); /* [0] => H3llö [1] => Wörld */
Working with Strings: