How comparison actually made
Each character has a numerical ASCII value, you can use the ord()
function to get the value for a character. For example, the ASCII values for the uppercase alphabet A-Z
are in the range of 65-90
, the lowercase alphabet a-z
are in the range 97-122
, and the numbers 0-9
are in the range 48-57
. See the following table:
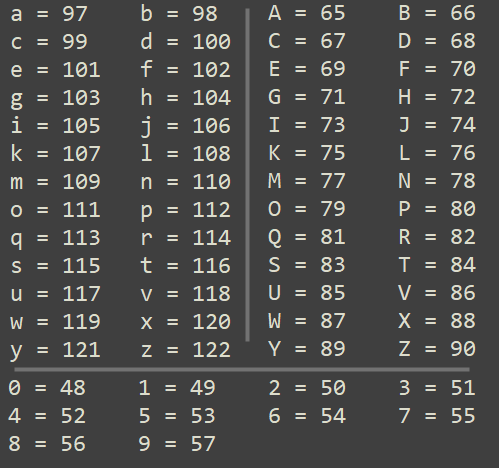
The ASCII value of a
is 97 and ASCII value of A
is 65, we see that 97
is greater than the 65
. When we compare a
and A
with strcmp
function, it returns < 0
if the first argument is less than the second argument, > 0
if the first argument is greater than the second argument, and 0
if they are equal.
<?php echo strcmp('a', 'A'); //Prints '1' echo strcmp('A', 'a'); //Prints '-1' echo strcmp('a', 'a'); //Prints '0'
If a string contains multiple characters then each character is compared in sequence. For example, comparing ab
and AB
, the first character a
ASCII value is 97
which is greater than the character a
ASCII value 65
, so the strcmp
function would return 1
, as the first argument is greater than the second.
<?php echo strcmp('ab', 'AB'); //Prints '1'
If the first character in the first argument is greater (or less) than the first character in the second argument, the other characters comparison is irrelevant. But what if a string contains numbers? In this situation, you must use the strnatcmp
and strnatcasecmp
functions.
strnatcmp
<?php //Syntax strnatcmp(string $string1, string $string2): int
This function returns:
< 0
ifstring1
is less thanstring2
,> 0
ifstring1
is greater thanstring2
, and0
ifstring1
andstring2
are equal.
This function compares characters in two strings using the ASCII values, but if there are any numbers within the string they are compared in the natural order. For example, in natural order 19 is greater than 9 so the USD 19
evaluates to greater than USD 9
. This function reads the numeric value regardless of its position in the string.
<?php echo strcmp('USD 10', 'USD 5'); //Prints '-1' echo strnatcmp('USD 10', 'USD 5'); //Prints '1'
strnatcasecmp
<?php //Syntax strnatcasecmp(string $string1, string $string2): int
This function works similar to the strnatcmp
function except it is case-insensitive.
<?php echo strnatcmp('USD 10', 'usd 5'); //Prints '-1' echo strnatcasecmp('USD 10', 'usd 5'); //Prints '1'
Working with Strings: