<?php mail($to, $subject, $message, $headers);
Once a form has been submitted, a notification can be implemented by sending the form data via email.
Sending Form Data Via Email
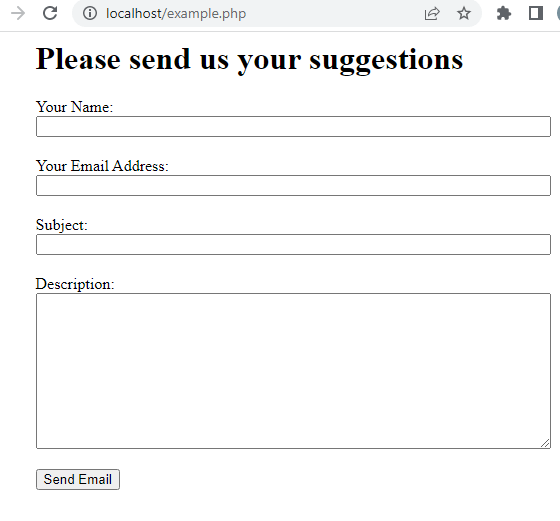
Creating HTML form for sending emails:
<html> <head><title>Email form</title> <style> form div {margin-bottom:10px; width:515px; margin: 0 auto 20px} input, textarea{width:100%} </style> </head> <body> <form method="post" action="example.php"> <div><h1>Please send us your suggestions</h1></div> <div> Your Name: <input type="text" name="name" required> </div> <div> Your Email Address: <input type="email" name="email" required> </div> <div> Subject: <input type="text" name="subject" required> </div> <div> Description:<br> <textarea name="body" rows="10" required></textarea> </div> <div> <button type="submit" name="submit">Send Email</button> </div> </form> </body></html>
Emailing form data:
<?php if ($_SERVER['REQUEST_METHOD'] == 'POST') { // See How to Validate Forms $to = $_POST['name'] . "<{$_POST['email']}>"; $subject = $_POST['subject']; $body = $_POST['body']; $headers = "From: BrainBell <info@brainbell.com>\r\n" . "X-Sender: <info@brainbell.com>\r\n" . "X-Mailer: PHP\r\n" . "X-Priority: 1\r\n" . "Return-Path: <info@brainbell.com>\r\n"; mail($to, $subject, $body, $headers); }
The preceding code creates the destination address $to
, the subject, the body, and additional headers of an email message, and then send that email message.
The $t
o address is created using the name, and email address of the visitor. It has the following format:
Complete Name <visitor@email.address.com>
How to send Cc and Bcc
Use the mail function’s additional header parameter to send the Ccc and Bcc emails, the headers should be separated with \r\n
:
<?php $to = $_POST['name'] . "<{$_POST['email']}>"; $subject = $_POST['subject']; $body = $_POST['body']; $headers = "From: BrainBell <info@brainbell.com>\r\n" . "X-Sender: <info@brainbell.com>\r\n" . "Bcc: admin@brainbell.com\r\n" . "Cc: brainbell@admin\r\n"; mail($to, $subject, $body, $headers);
Sending HTML Formatted Emails
You can send HTML emails by sending the additional Content-type and MIME-Version headers:
$headers[] = 'MIME-Version: 1.0';
$headers[] = 'Content-type: text/html; charset=iso-8859-1';
Example: Sending HTML mails in PHP:
<?php if ($_SERVER['REQUEST_METHOD'] == 'POST') { // Validate form (skipped in this tutorial) $to = $_POST['name'] . "<{$_POST['email']}>"; $subject = $_POST['subject']; $body = "<html><head> <title>The Suggestion Form</title> </head><body> <h1>$subject</h1> <p>".nl2br($_POST['body'])."</p> </body></html>"; $headers = "From: BrainBell <info@brainbell.com>\r\n" . "MIME-Version: 1.0\r\n" . "Content-type: text/html; charset=UTF-8\r\n" . "X-Sender: <info@brainbell.com>\r\n" . "X-Mailer: PHP\r\n" . "X-Priority: 1\r\n" . "Return-Path: <info@brainbell.com>\r\n"; mail($to, $subject, $body, $headers); }
Processing Forms in PHP: