Before attempting to use variables that come from an external source such as a user-submitted form, you must check whether those variables are defined or not. isset() function is one of the most common tests to check whether a variable has been defined.
Testing, setting, and unsetting variables
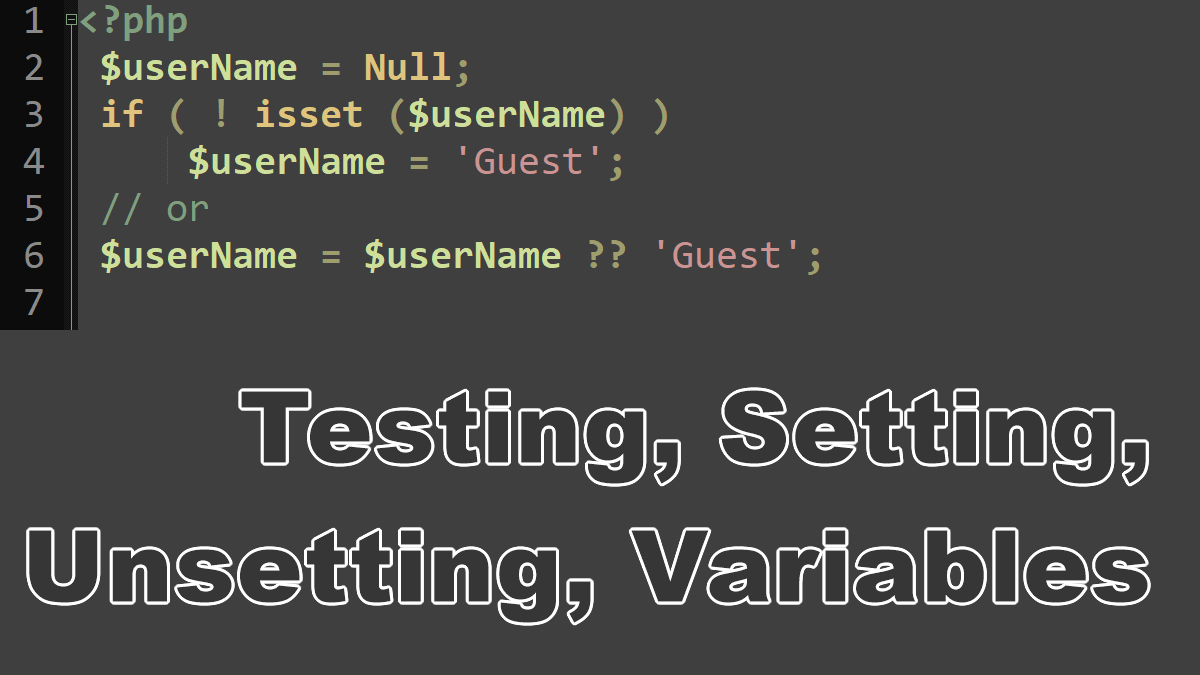